Embed App Builder Web SDK in a VueJS Web App
The following guide describes the process of embedding the App Builder Web SDK in a VueJS web app.
BUILDING
STEP 1
You need to download and extract the app builder source code, you can read more here
Run the app-builder-cli in your project folder using the following command:
npm i && npm run start
STEP 2
Navigate using the arrow keys and select the build option using the enter key
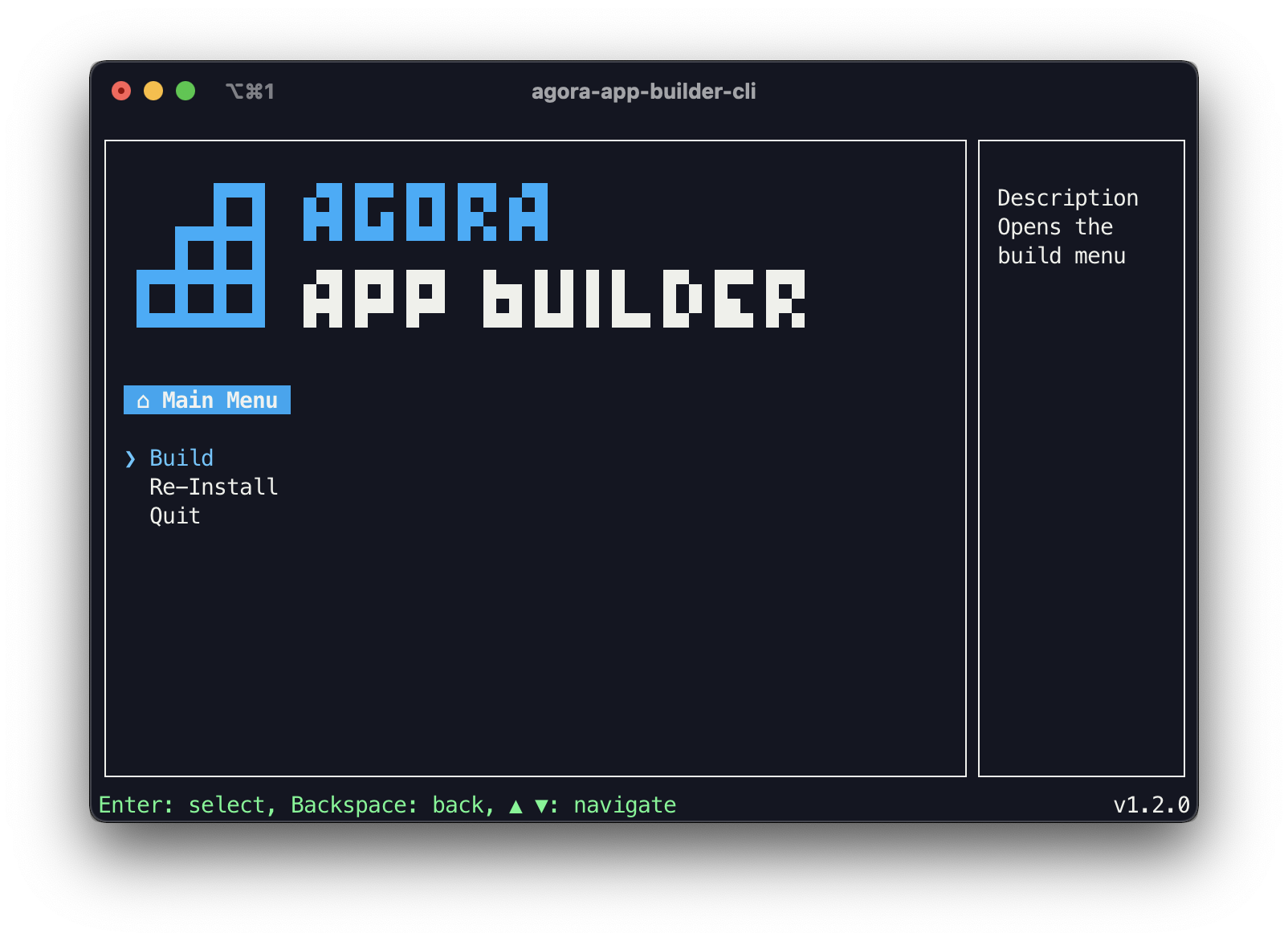
STEP 3
Select the Web SDK platform, after which the CLI will start the build process. This will take a few minutes.
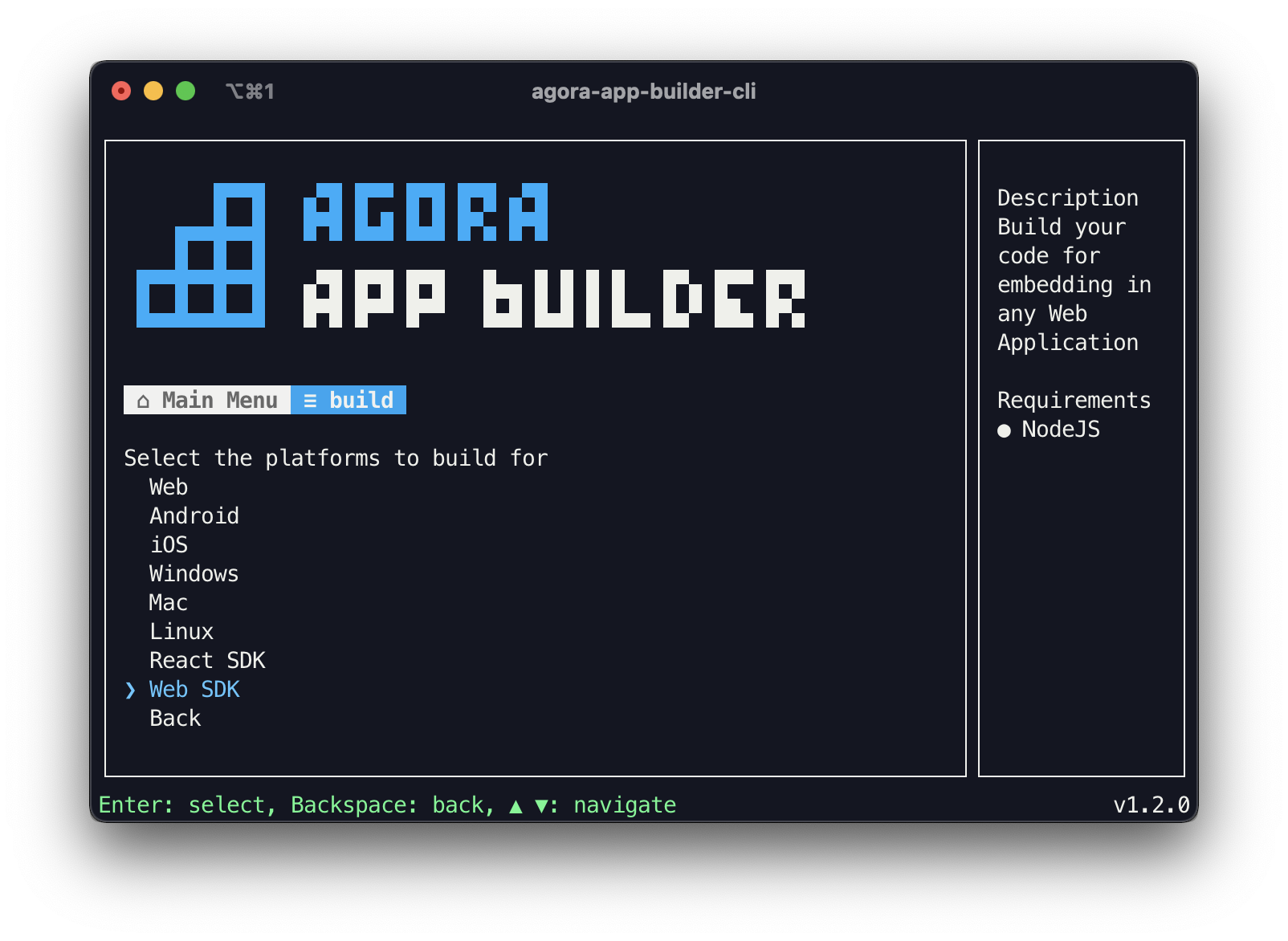
STEP 4
Select the Web SDK platform, after which the CLI will start the build process. This will take a few minutes.
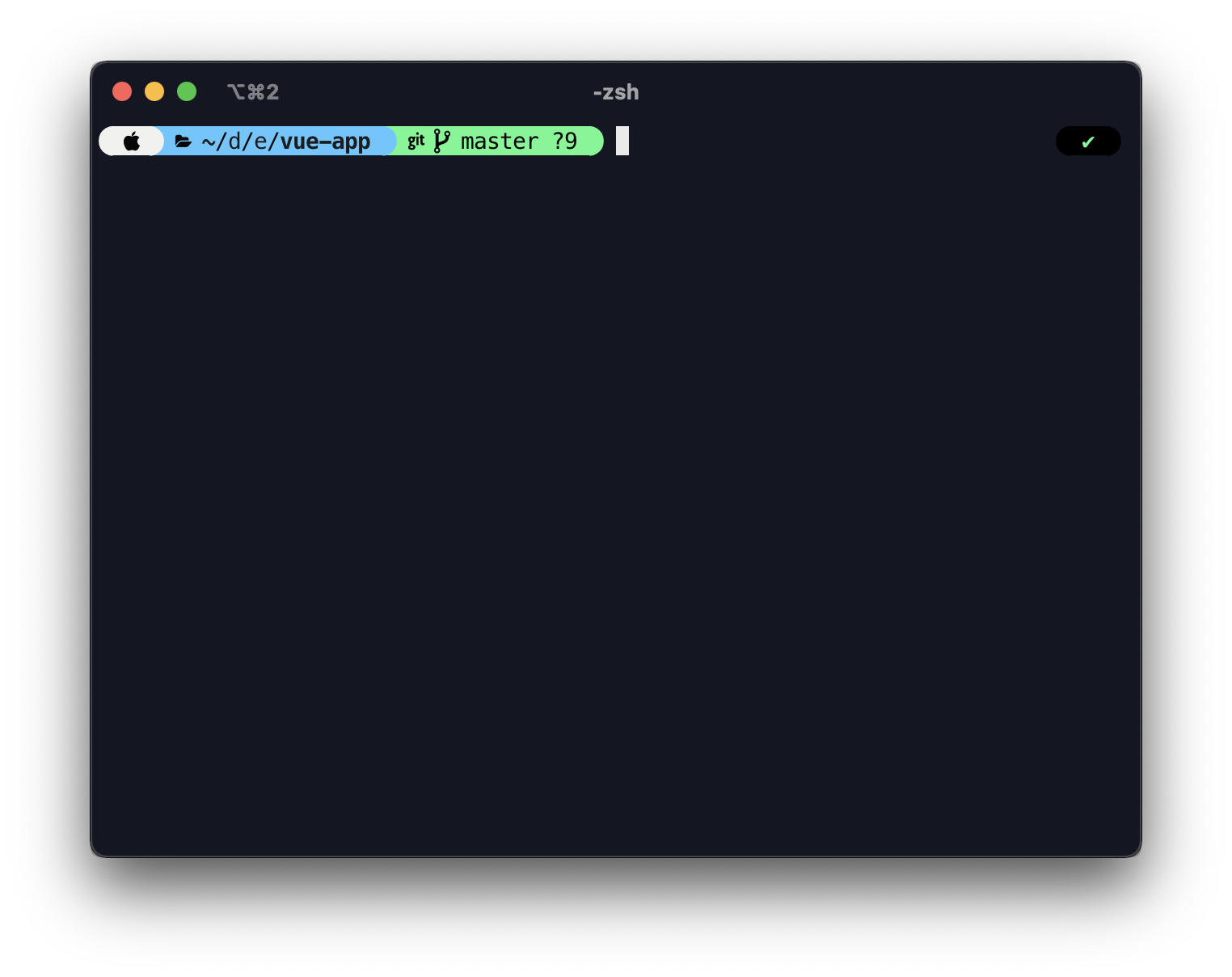
STEP 5
Navigate to <Agora App Builder>/Builds/web-sdk
and copy the wasm folder , png and ttf files into your project's public assets folder.
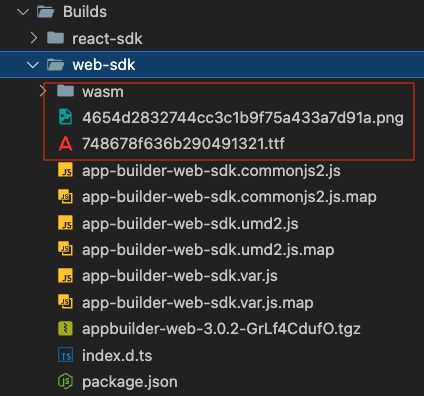
Now, import the font in the public/index.html
of the customized app.
<style type="text/css">
@font-face {
font-family: "Icomoon";
src: url(./static/fonts/748678f636b290491321.ttf) format("truetype");
}
</style>
USAGE
STEP 1
The module is installed with same name as your App Builder project. Import it just like any other node module into your desired Vue component.
<script>
import AgoraAppBuilder from "@appbuilder/web";
</script>
STEP 2
After importing the module your App Builder project can be rendered using the
Make sure to provide necessary styling including a width and height on the parent element of the custom html element for proper scaling.
<template>
<div style="width: 100vw; height: 550px;">
<app-builder />
</div>
</template>
STEP 3
Start the development server inside your VueJS project directory with the following command
npm run serve
STEP 4
You should now see your App Builder project being displayed in your desired Vue component.
STEP 5
The app-builder-cli compiles your App Builder project into a node module inside the <Agora App Builder>/Builds/web-sdk
folder. Simply install this module using the following command.
npm install <path-to-app-builder-project-folder>/Builds/web-sdk
CUSTOMIZATION
You can use Customization APIs to customize your embedded App Builder project.
Read this guide for more information
STEP 1
To create a Customization you need to access the createCustomization
method on the imported AgoraAppBuilder
object, which takes the UserCustomizationConfig
as a parameter and returns a customization object.
<script>
import AgoraAppBuilder from "@appbuilder/web";
const customization = AgoraAppBuilder.createCustomization({
/*
My Customization Config. See https://appbuilder-docs.agora.io/customization-api/quickstart to get started with customizing!
*/
});
</script>
STEP 2
Pass the returned customization object to the customize
method available under the same AgoraAppBuilder
object to apply the config to your embedded App Builder project.
<script>
import AgoraAppBuilder from "@appbuilder/web";
const customization = AgoraAppBuilder.createCustomization({
/*
My Customization Config. See https://appbuilder-docs.agora.io/customization-api/quickstart to get started with customizing!
*/
});
AgoraAppBuilder.customize(customization);
</script>
STEP 3
You should now be able to see your customizations in action!
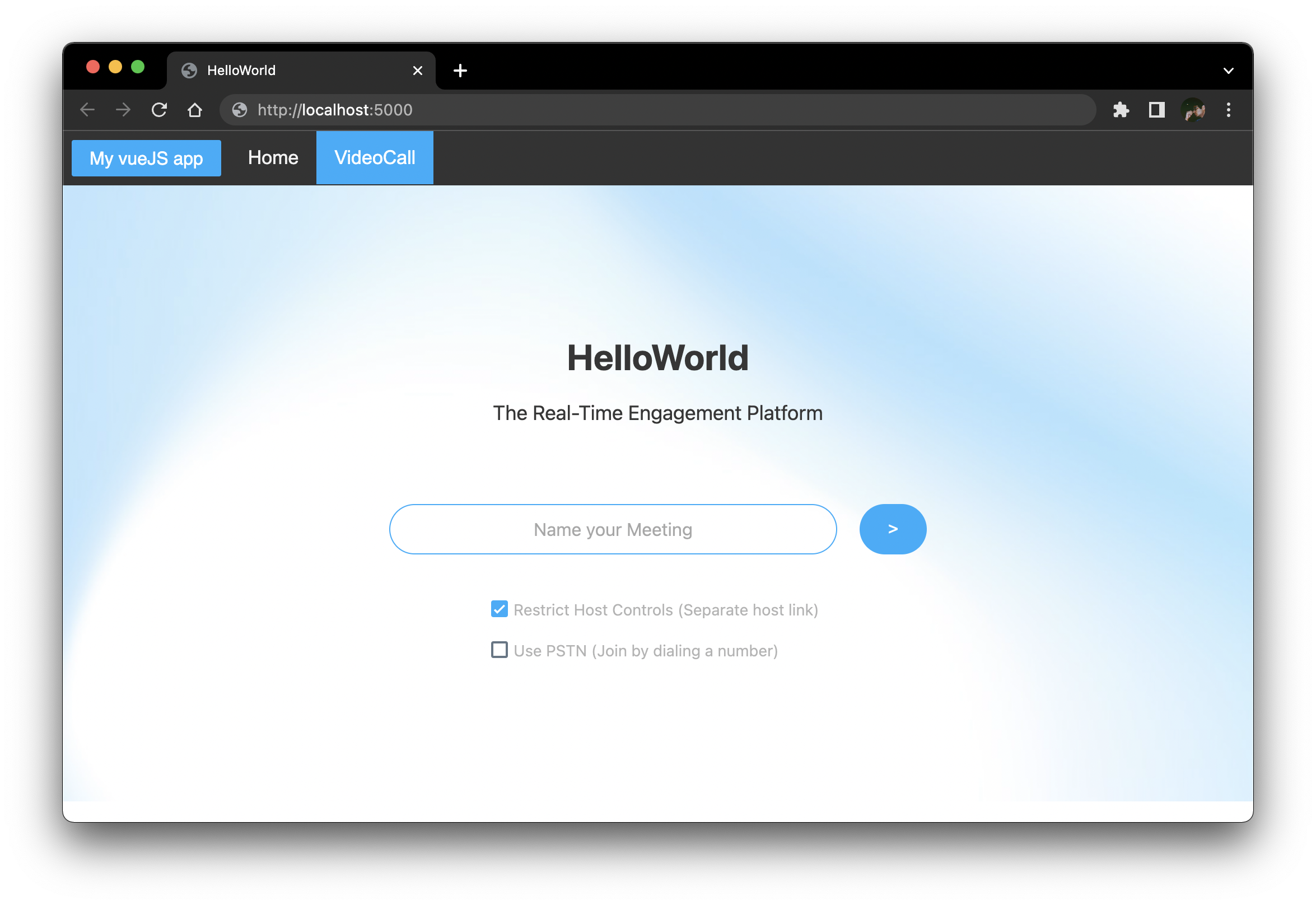