Using App builder APIs for your application
Introduction
To use these APIs, you first need an App builder JWT. To get these JWTs, you can configure your project in three ways in the app builder console to use these APIs.
- Default: Anyone can use your app. This gives an App builder JWT to all users who use the app. It is highly recommended that you use one of the other flows for your app.
- IDP: By setting up identity providers and configuring them in App builder console. This uses the user details returned by the identity provider to generate the JWT.
- Custom: This flow is intended to be called from app backends. If your use case involves maintaining user details
in-house or you have additional logic with identity providers, you can call
/v1/token/generate
from your app's backend to generate your user's JWT. To use realtime APIs with super-sdk, you need to use this custom flow.
Getting started
Default flow
Login to https://appbuilder.agora.io and create your new project in the console. If you go to the authentication section of your project, you can see that all newly created projects do not have any form of authentication enabled.
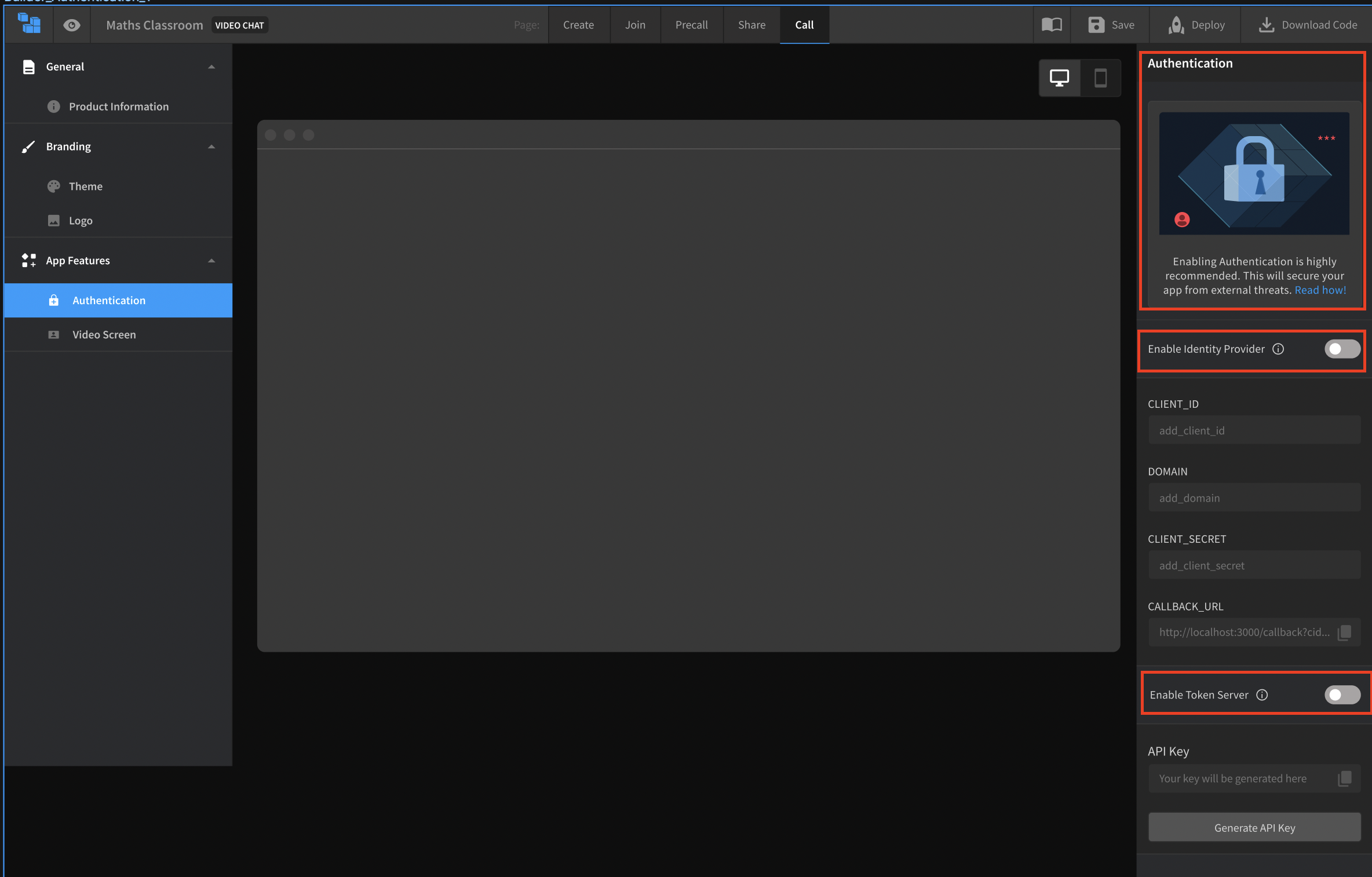
Call /login
as per these docs to generate a new JWT.
The returned JWT has to be sent in subsequent requests to other endpoints in the Authorization
header
as a Bearer
token.
IDP flow
Login to https://appbuilder.agora.io and open your project in the console and
Toggle Enable Identity Provider
as shown in the picture and fill in the relevant details.
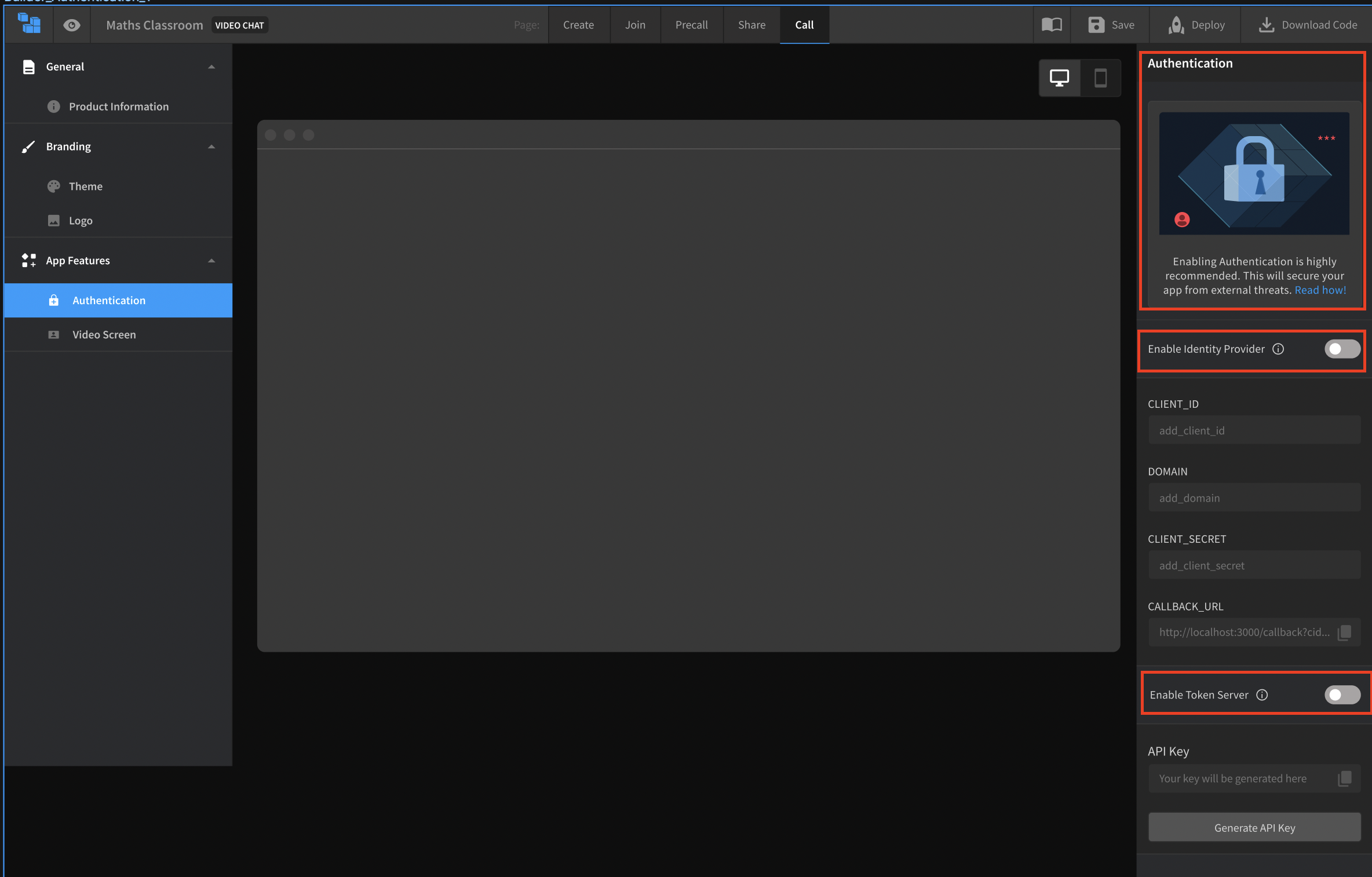
Call /idp/login
as per these docs to generate a new JWT.
In this flow, the JWT is set in the browser's cookies, so you can call other endpoints without any extra work.
Custom flow
Login to https://appbuilder.agora.io and open your project in the console and
Toggle Enable Token Server
and click on Generate API Key.
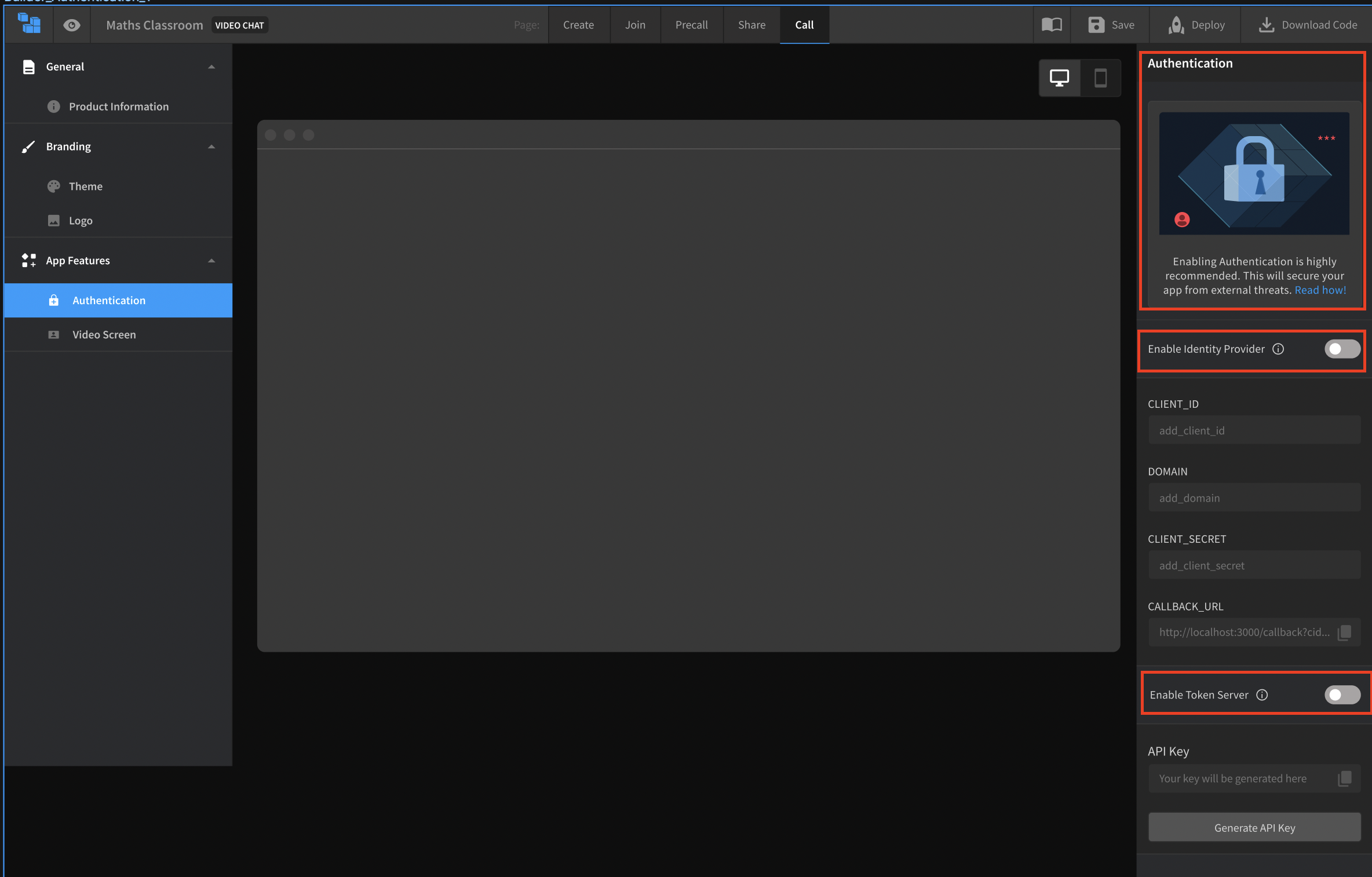
This returns an App builder project specific API key that is used by App builder APIs to verify that all requests are made for the particular project. This allows you to generate App builder JWTs on behalf of any user for your app.
Send a request to /v1/token/generate
as per these docs.
The returned JWT has to be sent in subsequent requests to other endpoints in the Authorization
header
as a Bearer
token.
Handling JWT tokens
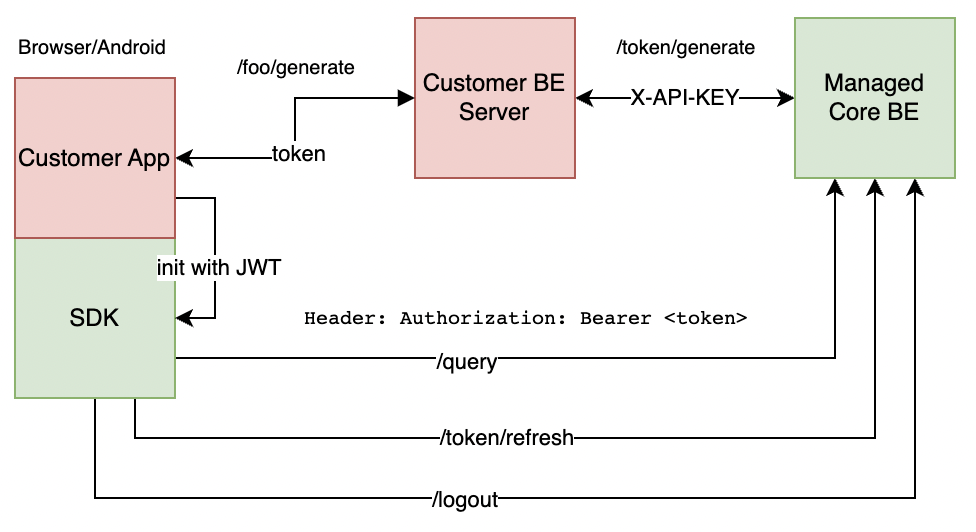
Token validity and refreshing token
The token generated in the getting started section is valid for 5 minutes. To ensure the user's client is not
locked out from using any API, send a request to /v1/token/refresh
as per these docs and repeat as necessary.
Logging a user out
To invalidate an App builder JWT before it expires, such as when a user logs out, send a request to the logout endpoint.
This endpoint is v1/idp/logout
for apps using the IDP login flow and /v1/logout
for apps using the custom login flow.
GraphQL APIs
We expose the same graphql APIs from the Heroku backend as before under the endpoint /v1/query
. For more details on
the APIs make a graphql introspection request like so (be sure to authenticate yourself for this curl to work)
curl 'https://managedservices-prod.rteappbuilder.com/v1/query' -X POST -H 'content-type: application/json' --data-raw '{"operationName":"IntrospectionQuery","variables":{},"query":"query IntrospectionQuery {\n __schema {\n queryType {\n name\n }\n mutationType {\n name\n }\n subscriptionType {\n name\n }\n types {\n ...FullType\n }\n directives {\n name\n description\n locations\n args {\n ...InputValue\n }\n }\n }\n}\n\nfragment FullType on __Type {\n kind\n name\n description\n fields(includeDeprecated: true) {\n name\n description\n args {\n ...InputValue\n }\n type {\n ...TypeRef\n }\n isDeprecated\n deprecationReason\n }\n inputFields {\n ...InputValue\n }\n interfaces {\n ...TypeRef\n }\n enumValues(includeDeprecated: true) {\n name\n description\n isDeprecated\n deprecationReason\n }\n possibleTypes {\n ...TypeRef\n }\n}\n\nfragment InputValue on __InputValue {\n name\n description\n type {\n ...TypeRef\n }\n defaultValue\n}\n\nfragment TypeRef on __Type {\n kind\n name\n ofType {\n kind\n name\n ofType {\n kind\n name\n ofType {\n kind\n name\n ofType {\n kind\n name\n ofType {\n kind\n name\n ofType {\n kind\n name\n ofType {\n kind\n name\n }\n }\n }\n }\n }\n }\n }\n}\n"}'
Using spaces and realtime API
This feature works with the super-sdk. To use this feature, it needs to be enabled on the App builder console.
TODO: no console design for setting enabling spaces and rate limit available yet
To use realtime features, you need to generate tokens for a specific space from
/v1/space/token/generate
docs. This works like/v1/token/generate
but it is specific to a space in a project.Using the JWT generated in step 1, you need to call
/v1/user/details
docs. This returns the pusher details to initialise super-sdk with and the channel and channel details for the user to publish to.Once the frontend super-sdk is initialised with pusher details obtained from step 2, you can use realtime API by sending events as mentioned in the [docs](
TODO: add link to super-sdk docs on how to construct and send events
) through pusher.