Embed App Builder Web SDK in an Angular Web App
The following guide describes the process of embedding the App Builder Web SDK in an Angular web app.
BUILDING
STEP 1
You need to download and extract the app builder source code, you can read more here.
Run the app-builder-cli in your project folder using the following command:
npm i && npm run start
STEP 2
Navigate using the arrow keys and select the Build
option using the enter key
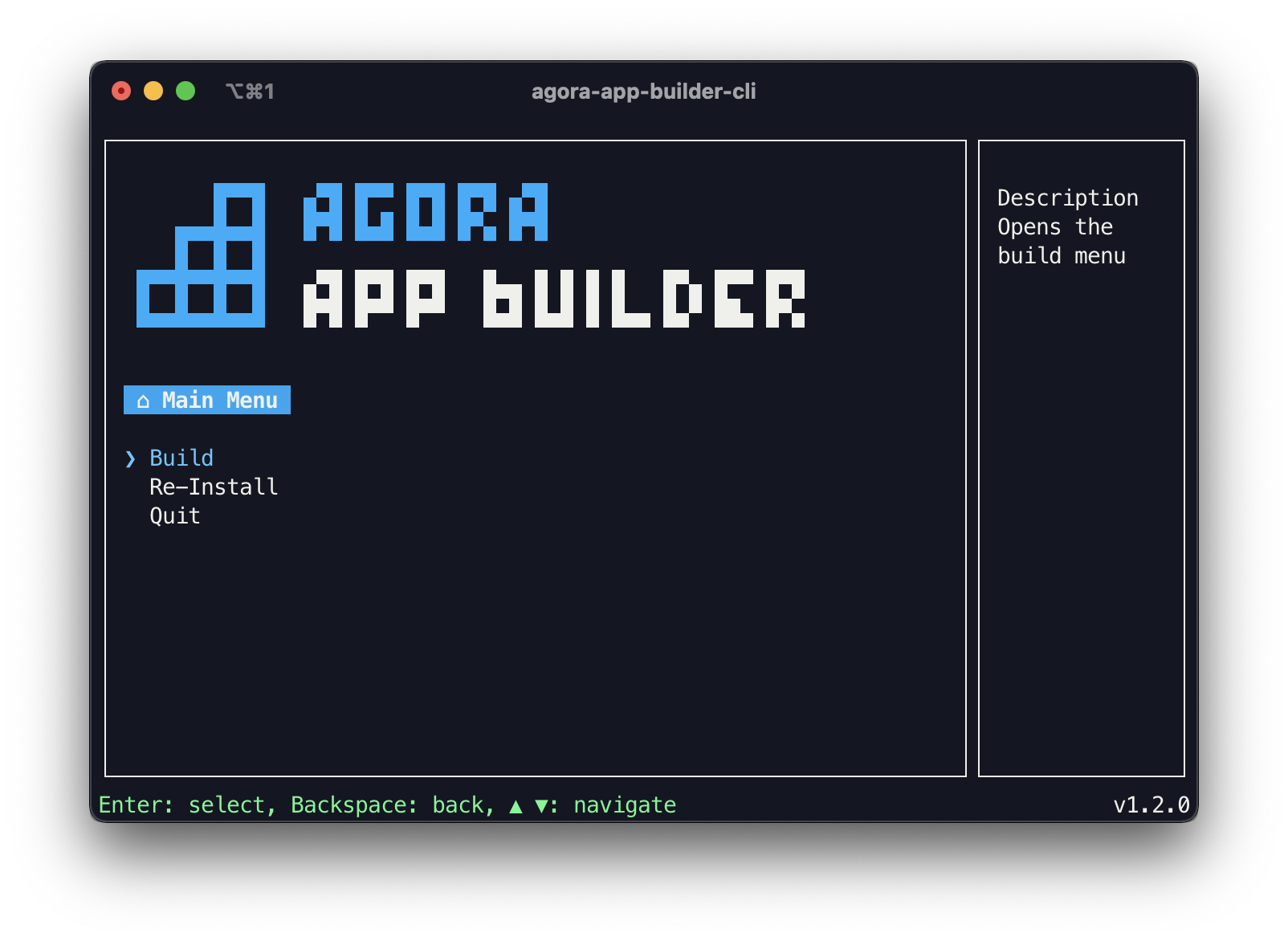
STEP 3
Select the Web SDK
platform, after which the CLI will start the build process. This will take a few minutes.
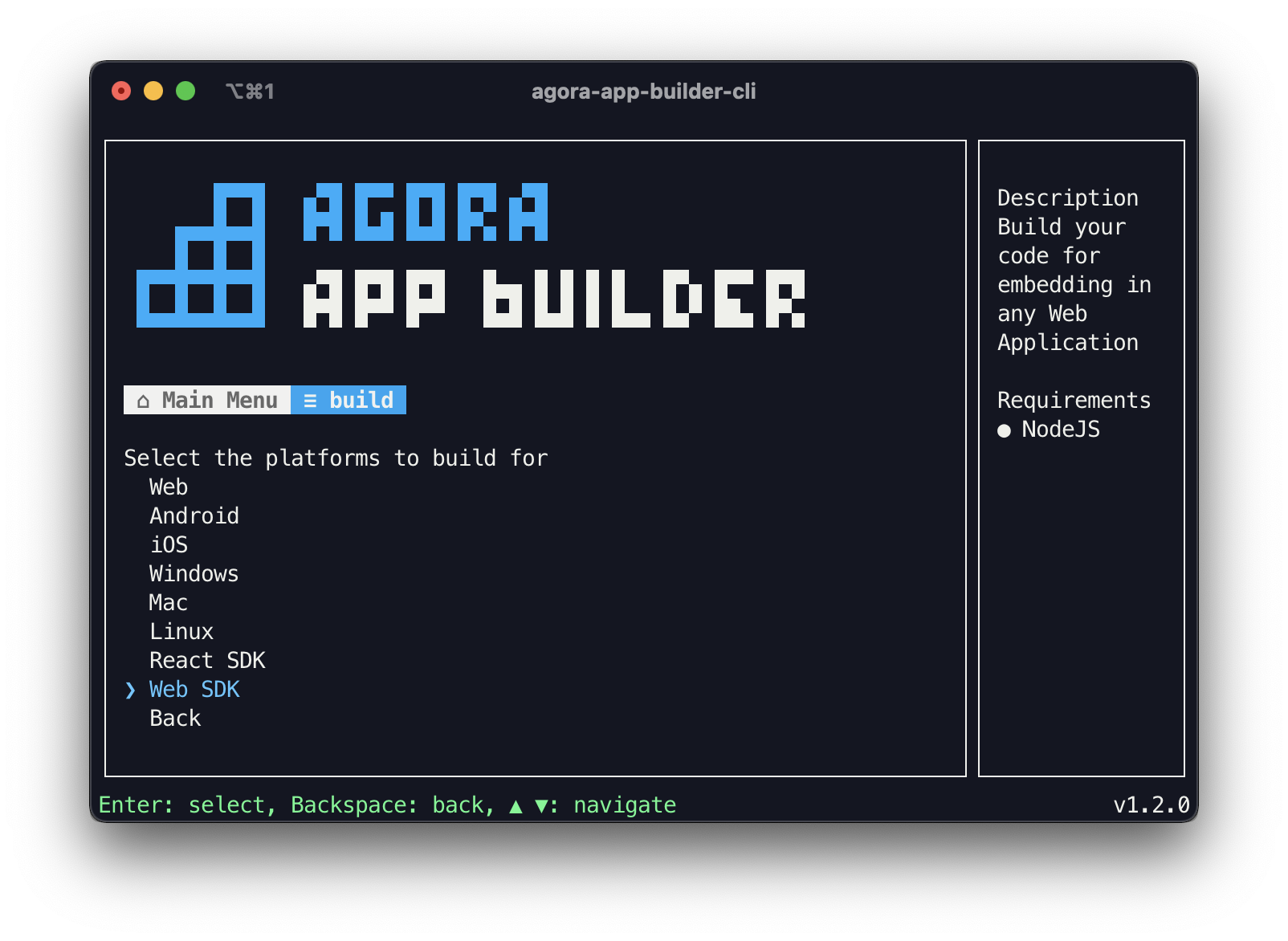
STEP 4
Once the build is complete open a terminal window in your Angular project directory.
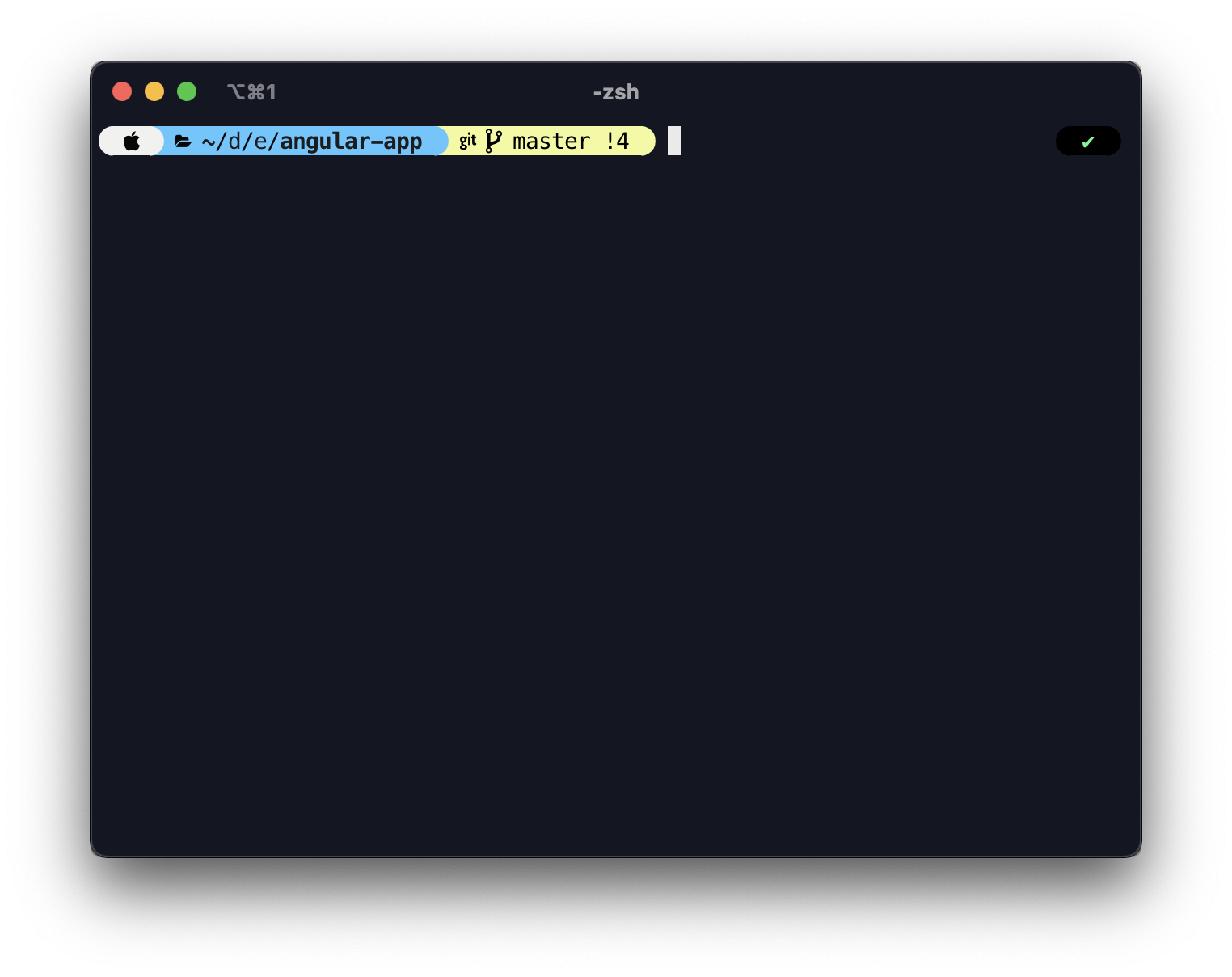
STEP 5
The app-builder-cli compiles your App Builder project into a node module inside the <Agora App Builder>/Builds/web-sdk
folder. Simply install this module using the following command.
npm install <path-to-app-builder-project-folder>/Builds/web-sdk
STEP 6
Navigate to <Agora App Builder>/Builds/web-sdk
and copy the wasm folder , png and ttf files into your project's public assets folder.
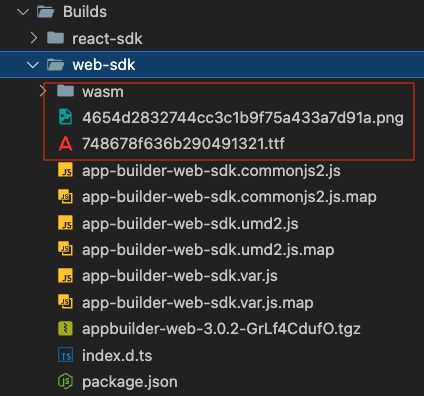
Now, import the font in the public/index.html
of the customized app.
<style type="text/css">
@font-face {
font-family: "Icomoon";
src: url(./static/fonts/748678f636b290491321.ttf) format("truetype");
}
</style>
USAGE
STEP 1
The module is installed with same name as your App Builder project. Import it just like any other node module into your desired angular component file.
import { Component } from "@angular/core";
import AgoraAppBuilder from "@appbuilder/web";
// To prevent removal of AgoraAppBuilder via dead code elimination.
console.log(AgoraAppBuilder);
STEP 2
After importing the module your App Builder project can be rendered using the <app-builder />
custom html element.
Make sure to provide necessary styling including a width and height on the parent element of the custom html element for proper scaling.
...
@Component({
selector: 'app-root',
template: ` <div id="app-builder-container">
<app-builder></app-builder>
</div> `,
styles: [`
#app-builder-container{
width: 100vw;
height: 100vh;
}
`],
})
...
STEP 3
You also need to add CUSTOM_ELEMENTS_SCHEMA
in your module file for your component to enable support for custom html elements.
import { NgModule, CUSTOM_ELEMENTS_SCHEMA } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
...
@NgModule({
declarations: [AppComponent],
imports: [BrowserModule],
providers: [],
bootstrap: [AppComponent],
schemas: [CUSTOM_ELEMENTS_SCHEMA],
})
...
STEP 4
Start the development server inside your Angular project directory with the following command
ng serve
STEP 5
You should now see your App Builder project being displayed in your desired Angular component.
CUSTOMIZATIONS
STEP 1
You can use Customization APIs to customize your embedded App Builder project.
Read this guide for more information.
STEP 2
To create a Customization you need to access the createCustomization
method on the imported AgoraAppBuilder
object, which takes the UserCustomizationConfig
as a parameter and returns a customization object.
import AgoraAppBuilder from "@appbuilder/web";
const customization = AgoraAppBuilder.createCustomization({
/*
My Customization Config. See https://appbuilder-docs.agora.io/customization-api/quickstart to get started with customizing!
*/
});
STEP 3
Pass the returned customization object to the customize
method available under the same AgoraAppBuilder
object to apply the config to your embedded App Builder project.
import AgoraAppBuilder from "@appbuilder/web";
const customization = AgoraAppBuilder.createCustomization({
/*
My Customization Config. See https://appbuilder-docs.agora.io/customization-api/quickstart to get started with customizing!
*/
});
AgoraAppBuilder.customize(customization);
STEP 4
You should now be able to see your customizations in action!
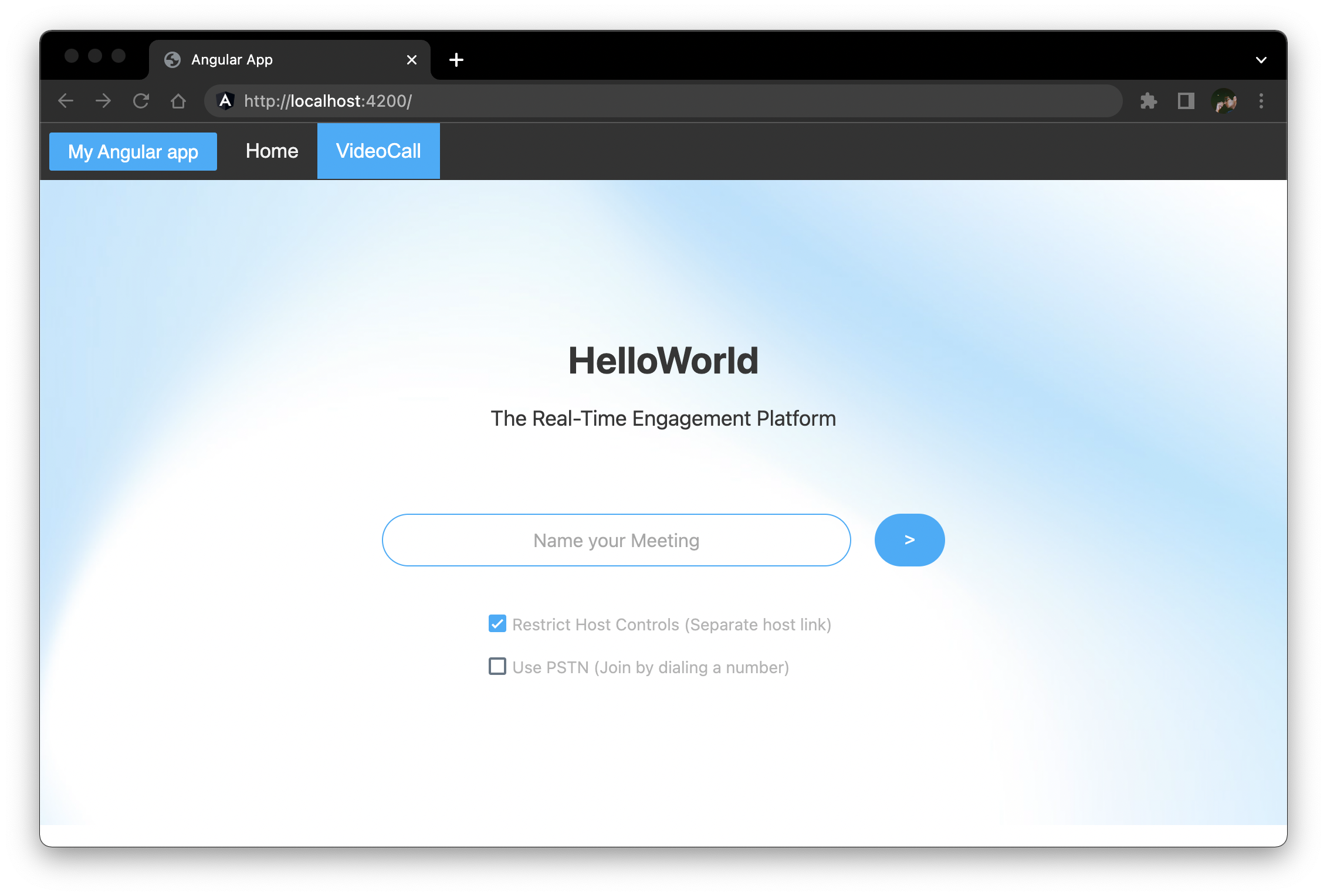