Embed App Builder Web SDK in a NextJS Web App
The following guide describes the process of embedding the App Builder React SDK in a NextJS app.
Building
STEP 1
You need to download and extract the app builder source code, you can read more here.
Run the app-builder-cli in your project folder using the following command:
npm i && npm run start
STEP 2
Navigate using the arrow keys and select the Build
option using the enter key
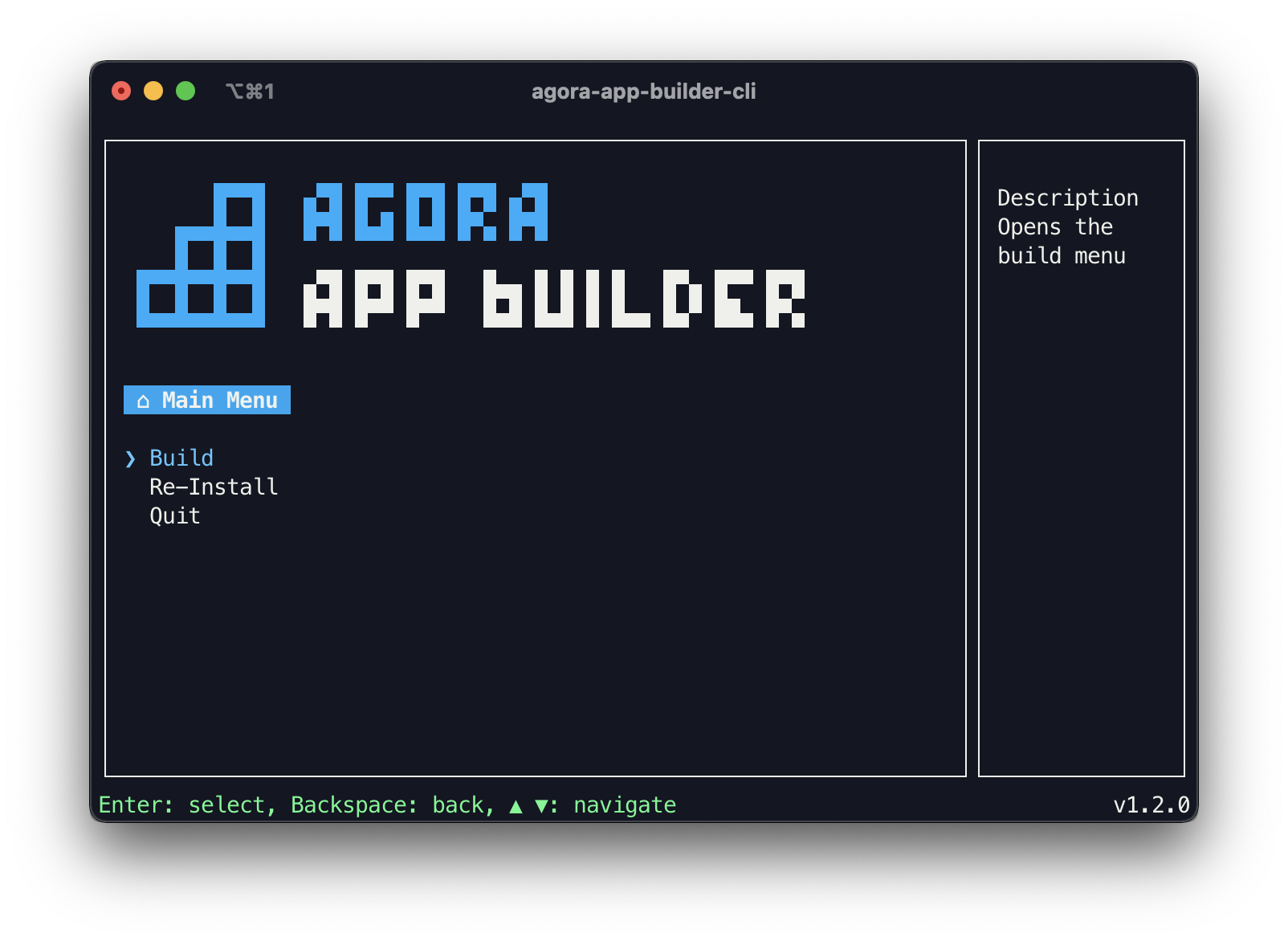
STEP 3
Select the React SDK
platform, after which the CLI will start the build process. This will take a few minutes.
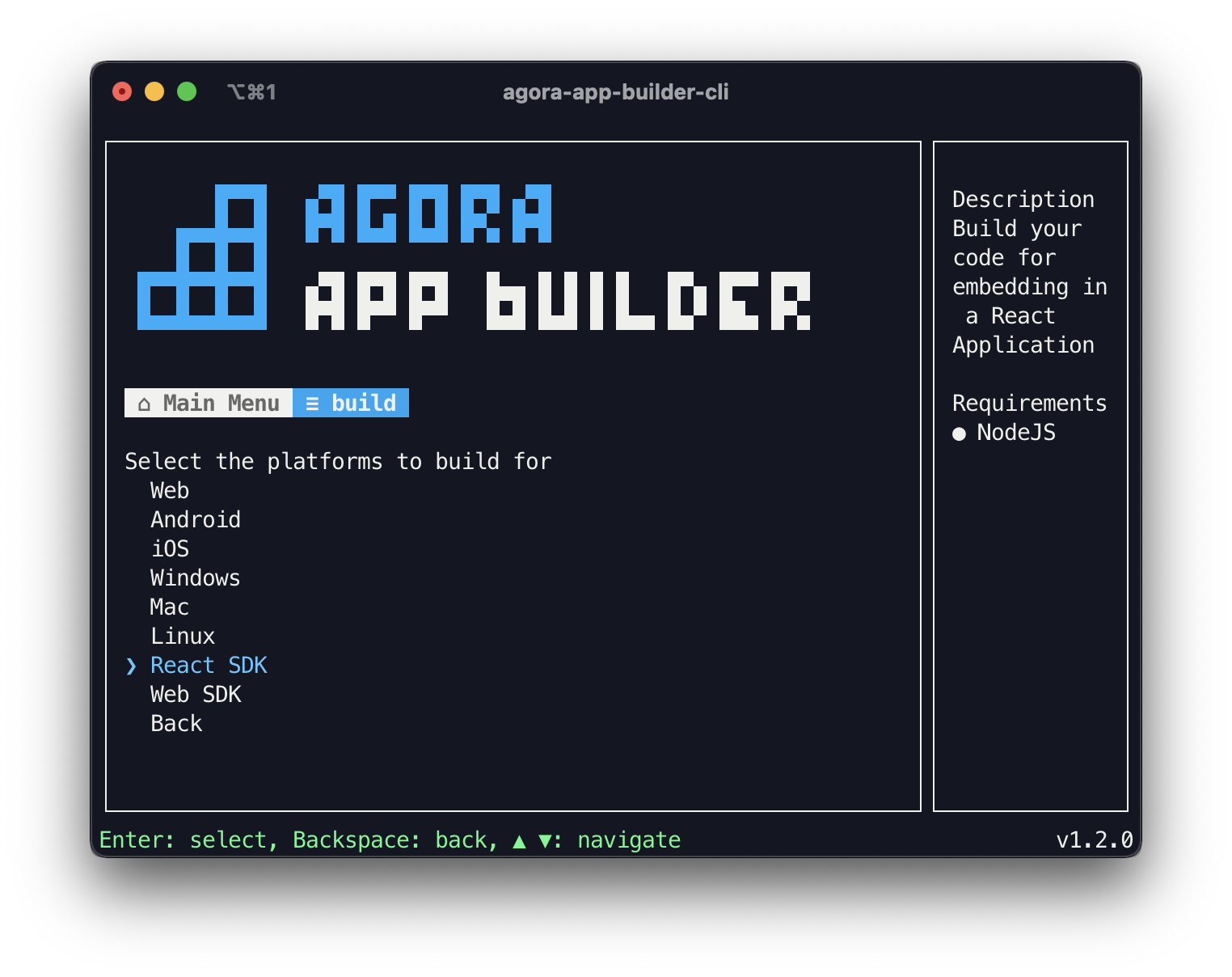
STEP 4
Once the build is complete open a terminal window in your NextJs project directory.
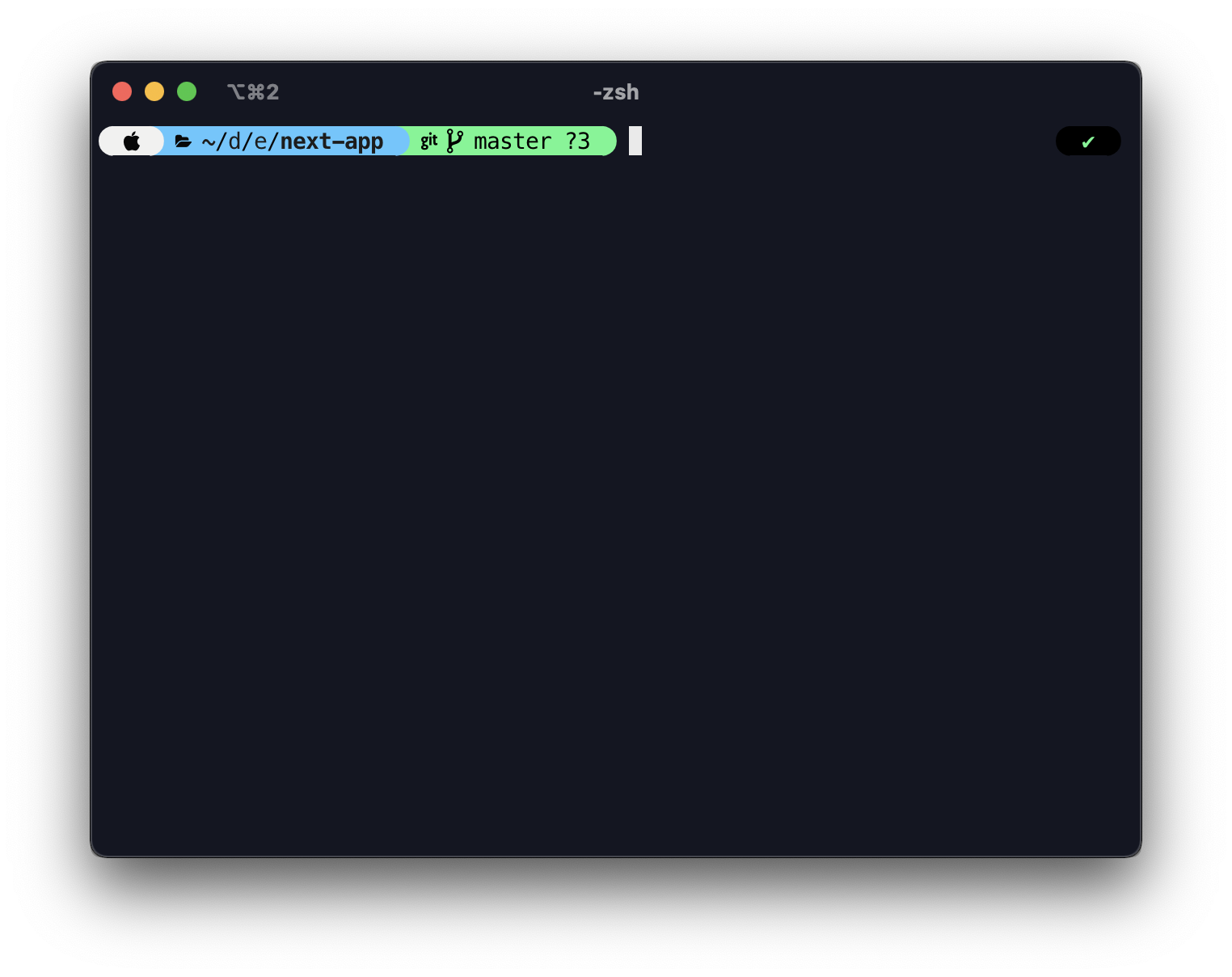
STEP 5
The app-builder-cli compiles your App Builder project into a node module inside the <Agora App Builder>/Builds/react-sdk
folder. Simply install this module using the following command.
npm install <path-to-app-builder-project-folder>/Builds/react-sdk
STEP 6
Navigate to <Agora App Builder>/Builds/react-sdk
and copy the wasm folder , png and ttf files into your project's public assets folder.
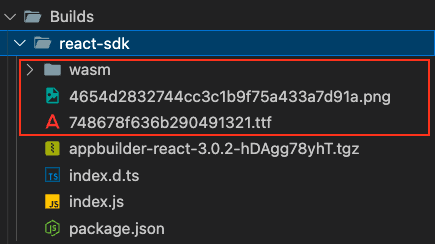
Now, import the font in the public/index.html
of the customized app.
<style type="text/css">
@font-face {
font-family: "Icomoon";
src: url(./static/fonts/748678f636b290491321.ttf) format("truetype");
}
</style>
Usage
STEP 1
The module is installed with same name as your App Builder project which can be imported into your Nextjs App.
However in a NextJS environment, Client Side rendering must be enforced for the App Builder component. One way to do so is by importing App Builer into a new App Builder wrapper component.
import AgoraAppBuilder from "@appbuilder/react";
STEP 2
After importing the module your App Builder project can be rendered using the View
property on the imported AgoraAppBuilder
object.
Make sure to provide necessary styling including a width, height and display flex on the parent element of the View
component for proper scaling.
import AgoraAppBuilder from "@appbuilder/react";
const AppBuilderWrapper = () => {
return (
<div style={{ display: "flex", width: "100vw", height: "550px" }}>
<AgoraAppBuilder.View />
</div>
);
};
STEP 3
Dynamically import the wrapper in your desired Nextjs page/component using the dynamic
function.
import dynamic from "next/dynamic";
const AgoraAppBuilderWraper = dynamic(
() => import("../components/AppBuilderWrapper"),
{
ssr: false,
},
);
STEP 4
After dynamically importing the wrapper it can be rendered normally in any page/component.
You can add a suppressHydrationWarning={true}
prop to the parent element of the wrapper to suppress any subsequent hydration warnings emitted due to client side rendering of its children component.
import dynamic from "next/dynamic";
const AgoraAppBuilderWraper = dynamic(
() => import("../components/AppBuilderWrapper"),
{
ssr: false,
},
);
const VideoCall = () => {
return (
<div suppressHydrationWarning={true}>
<AgoraAppBuilderWrapper />
</div>
);
};
STEP 5
Start the development server inside your NextJs project directory with the following command
npm run dev
STEP 6
You should now see your App Builder project being displayed in your desired web page.
Customization
STEP 1
You can use Customization APIs to customize your embedded App Builder project.
Read this guide for more information.
STEP 2
To create a Customization you need to access the createCustomization
method on the imported AgoraAppBuilder
object, which takes the UserCustomizationConfig
as a parameter and returns a customization object.
Make sure you do this on component mount for best results.
import AgoraAppBuilder from "@appbuilder/react";
const AppBuilderWrapper = () = {
useEffect(()=>{
const customization = AgoraAppBuilder.createCustomization({
/*
My Customization Config. See https://appbuilder-docs.agora.io/customization-api/quickstart to get started with customizing!
*/
});
},[])
...
STEP 3
Pass the returned customization object to the customize
method available under the same AgoraAppBuilder
object to apply the config to your embedded App Builder project.
import AgoraAppBuilder from "@appbuilder/react";
const AppBuilderWrapper = () = {
useEffect(()=>{
const customization = AgoraAppBuilder.createCustomization({
/*
My Customization Config. See https://appbuilder-docs.agora.io/customization-api/quickstart to get started with customizing!
*/
});
AgoraAppBuilder.customize(customization);
},[])
...
STEP 4
You should now be able to see your customizations in action!